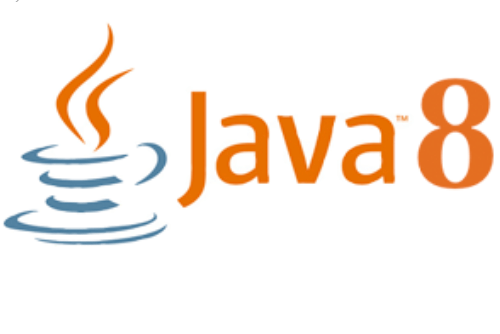
Java 8 Streams – Create a map from a collection, list or set
Preamble
With the introduction of streams in Java 8 the writing of Java code has changed. With Java 8 you can now transfer objects from a list to a map in a comfortable way. How to implement this I will show you in this tutorial.
Requirement
- Java knowledge
Convert Collection, List or Set to Map with the Java 8 Streaming API
As a developer you sometimes face the problem that objects of a collection, list or set have to be transferred into a map. There are different reasons for this. One reason might be that the access to the elements of a map is much faster than the access to the elements of a list. In this case it makes sense to transfer the collection, list or set into a map in advance.
In this tutorial we assume that we have a list of the class Customer.java and that it has the properties id, firstname, lastname.
package de.ertantoker.tutorial.model; public class Customer { private String id; private String firstname; private String lastname; public Customer(String id, String firstname, String lastname) { this.id = id; this.firstname = firstname; this.lastname = lastname; } public String getId() { return id; } public void setId(String id) { this.id = id; } public String getFirstname() { return firstname; } public void setFirstname(String firstname) { this.firstname = firstname; } public String getLastname() { return lastname; } public void setLastname(String lastname) { this.lastname = lastname; } }
With the Streamin API of Java 8 we can use streams and at the end of the processing we can transfer them via collectors into a target structure suitable for us. In this example we want to convert from a list to a map.
The following code shows how to transfer a List<Customer> to Map<String, Customer>.
package de.ertantoker.tutorial; import de.ertantoker.tutorial.model.Customer; import java.util.Arrays; import java.util.List; import java.util.Map; import java.util.stream.Collectors; public class Java8ListToMap { public static void main(String[] args) { Customer customer1 = new Customer("1", "Albert", "Einstein"); Customer customer2 = new Customer("2", "Otto", "Hahn"); Customer customer3 = new Customer("3", "Isaac", "Newton"); List customerList = Arrays.asList(customer1, customer2, customer3); Map<String, Customer> customerMap = customerList .stream() .collect(Collectors.toMap(Customer::getId, customer -> customer)); } }
The Result
We have now learned how to convert lists or sets into a map with Java 8 and Streams Collection. With the collector classes it is also possible to convert the list into a set or to convert the objects in the list into another class. The Streams API offers a lot of possibilities. How to convert the objects of a list into another class you can see in my next tutorial Java 8 Stream Object mapping.
I hope you enjoyed my little tutorial. If you should have questions, criticism or suggestions, then I would be pleased, if you would leave a comment.